Some web space to sketch, doodle and make notes. Made using these tools. See more of my work here.
void ofApp::updateAnim(){
for(int i = 0; i < 12; i++) {
createMover();
}
if(ofGetFrameNum() == 40) {
trails = true;
}
if(ofGetFrameNum() == 80) {
running = false;
stopping = true;
}
if(ofGetFrameNum() == 94) {
stopping = false;
trails = false;
}
if(ofGetFrameNum() == 98 || ofGetFrameNum() == 108) {
resetting = !resetting;
}
if(ofGetFrameNum() == 110) {
save = true;
}
for(int i = 0; i < movers.size(); i++) {
movers.at(i).applyForce(gravity);
movers.at(i).update();
}
}
void ofApp::drawAnim() {
if(!trails) {
ofSetHexColor(0x100643);
ofRect(0, 0, width, height);
}
if(running) {
for(int i = 0; i < movers.size(); i++) {
movers.at(i).draw();
}
}
if(stopping) {
ofSetColor(ofColor::white);
font.drawString("STOP", 70, 120);
}
if(resetting) {
ofSetColor(ofColor::white);
font.drawString("RESET", 64, 120);
}
if(save) {
renderGif();
movers.clear();
save = !save;
}
}
void ofApp::createMover() {
minX = width * 0.4;
maxX = width * 0.6;
x = ofRandom(minX, maxX);
minY = height * 0.1;
maxY = height * 0.7;
y = ofRandom(minY, maxY);
color.setHsb(ofMap(y, minY, maxY, 0, 50), 255, 128);
initial = ofVec2f(ofMap(x, minX, maxX, -3, 3), 0);
PatternMover mover;
mover.setup();
mover.setColor(color);
mover.setSize(3);
mover.applyForce(initial);
mover.setLocation(x, y);
movers.push_back(mover);
}
Toying with simple forces

void ofApp::setupAnim() {
ofDisableArbTex();
img.loadImage("tissue.png");
texture = img.getTextureReference();
texture.setTextureWrap(GL_MIRRORED_REPEAT, GL_MIRRORED_REPEAT);
offset = ofRandom(1);
shift = false;
numShifts = 0;
//Position the planes to take up the full screen
for(int i = 0; i < 2; i++) {
planes.push_back(plane);
planes.at(i).set(ofGetHeight(), ofGetWidth());
planes.at(i).setPosition(ofGetWidth() * 0.5, ofGetHeight() * 0.5, 0);
planes.at(i).setResolution(2, 2);
}
}
void ofApp::updateAnim(){
if(!shift && ofRandom(1) < 0.15) {
numShifts++;
shift = true;
offset = getNewOffset();
}
tx0 = 0 * offset * 0.1;
ty0 = offset + ofGetFrameNum() * 0.001;
tx1 = tx0 + 1;
ty1 = ty0 + 2;
//Position the texture on the plane
planes.at(0).mapTexCoords(tx0, ty0, tx1, ty1);
planes.at(1).mapTexCoords(tx1, ty1, tx0, ty0);
}
void ofApp::drawAnim() {
ofBackground(0, 0, 0);
if(shift) {
if(ofRandom(1) > 0.2) shift = false;
} else {
texture.bind();
for(int i = 0; i < planes.size(); i++) {
planes.at(i).draw();
}
texture.unbind();
}
}
float ofApp::getNewOffset() {
do {
candidateOffset = ofRandom(1);
} while(abs(candidateOffset - offset) < 0.2);
return candidateOffset;
}
Overlaid media with alpha transparency, glimmering when squished into 400 pixels and slowly translated.

void ofApp::drawGrid() {
for(int i = 0; i < width * 0.1 + 10; i++) {
for(int j = 0; j < height * 0.1 + 10; j++) {
noise.x = noiseOffset.x + i * scaleX;
noise.y = noiseOffset.y + j * scaleY;
noise.z = noiseOffset.z + sineOfTheTime;
noiseValue = ofNoise(noise.x, noise.y, noise.z);
//http://natureofcode.com/book/chapter-3-oscillation#35-polar-vs-cartesian-coordinates
theta = noiseValue * 2 * PI;
x = r * cos(theta);
y = r * sin(theta);
ofPushMatrix();
ofTranslate(i * 10, j * 10);
ofEnableBlendMode(OF_BLENDMODE_ADD);
if(j % 2 == 0){
ofSetColor(180, 231, 248, 150);
ofRect(0, 0, x, y);
} else {
ofSetColor(255, 180, 180, 128);
ofLine(0, 0, x, y);
}
ofDisableBlendMode();
ofPopMatrix();
}
}
}
Perlin noise coupled with a little polar to cartesian conversion.

void ofApp::setupAnim() {
current = 0;
max = 80;
for(int i = 0; i < max; i++) {
glitches.push_back(templateGlitch);
glitches.at(glitches.size()-1).allocate(width, height);
}
ofSetColor(ofColor::white);
}
void ofApp::drawAnim() {
if(ofGetFrameNum() % 5 == 0) {
ofClear(255,255,255);
} else {
ofEnableBlendMode(OF_BLENDMODE_MULTIPLY);
glitches.at(ofGetFrameNum()%max).draw(0, 0);
ofDisableBlendMode();
}
}
Accessing chunks of memory that you're not supposed to access
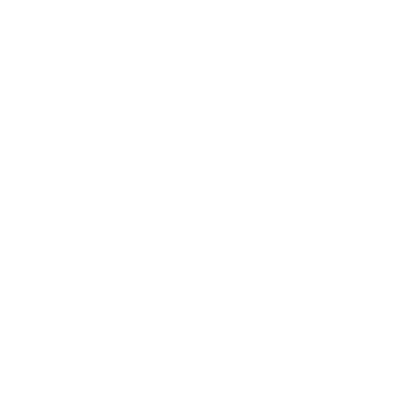
void ofApp::setupAnim() {
ofSetCircleResolution(100);
ofNoFill();
ofEnableSmoothing();
multiplier = 5;
switchDirection = false;
}
void ofApp::updateAnim(){
if(switchDirection) {
multiplier += 0.2;
} else {
multiplier -= 0.2;
}
if(multiplier <= 0.2 || multiplier >= 10) {
switchDirection = !switchDirection;
if(!switchDirection) saveNow = true;
}
}
void ofApp::drawAnim() {
ofBackground(0, 0, 0);
for(int i = 1; i <= 100; i++) {
ofCircle(width * 0.5, height * 0.5, i * multiplier);
ofCircle(width * 0.5, height * 0.5, i * (15 - multiplier));
}
if(!renderMode) {
ofDrawBitmapString(ofToString(multiplier), 20, 20);
}
}
Concentric circles, moving against each other. As rendered by the pixel grid of your monitor.

void ofApp::setupAnim() {
increment = 10;
max = 400;
pos = 0;
}
void ofApp::updateAnim(){
pos += increment;
if(pos > max * 2) {
pos = 0;
vertical = !vertical;
}
}
void ofApp::drawAnim() {
ofBackground(0, 0, 0);
for(int i = 1; i <= max; i++) {
if(vertical) drawVerticalLines(i);
else drawHorizontalLines(i);
}
}
void ofApp::drawVerticalLines(int x) {
if(x % 2 == 0) {
ofLine(x, pos - max, x, pos);
} else {
ofLine(x, height - pos, x, max * 2 - pos);
}
}
void ofApp::drawHorizontalLines(int y) {
if(y % 2 == 0) {
ofLine(pos - max, y, pos, y);
} else {
ofLine(height - pos, y, max * 2 - pos, y);
}
}
Something feels beautiful about working with the simplicity of lines.

void ofApp::drawAnim() {
ofBackground(0, 0, 0);
font.drawString("AN EMPTY\nGESTURE", 12, 85);
for(int i = 0; i < 30; i++) {
ofLine(ofRandom(520), ofRandom(200), ofRandom(520), ofRandom(200));
}
}
Sometimes you just need to start...
